Self-Improvement
기초 2 (클래스, 인스턴스, 접근제어, 변수, 해시(파이썬의 딕셔너리)) 본문
접근 제어
- Public 메소드는 누구나 호출할 수 있으며 기본적으로 Public으로 선언된다.
- Protected 메소드는 그 객체를 정의한 클래스와 하위 클래스에서만 호출할 수 있다.
- Private 메소드는 오직 현재 객체의 문맥 하에서만 호출할 수 있다.
각 접근제어를 선언 후에 작성 예제코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
class Test
def method1
p "public method1"
end
protected
def method2
p "protected method2"
end
private
def method3
p "private method3"
end
public
def method4
p "public method4"
end
end
|
cs |
선언 후의 접근제어 설정 예제코드
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
class Test
def method1
p "public method1"
end
def method2
p "protected method2"
end
def method3
p "private method3"
end
def method4
p "public method4"
end
public :method1, :method4
protected :method2
private :method3
end
|
cs |
변수
변수 출력 예제코드
변수는 클래스, ID, 값 등의 특징을 가지고 있다.
1
2
3
4
|
name="jo"
p "#{name.class}"
p "#{name.object_id}"
p "#{name}"
|
cs |
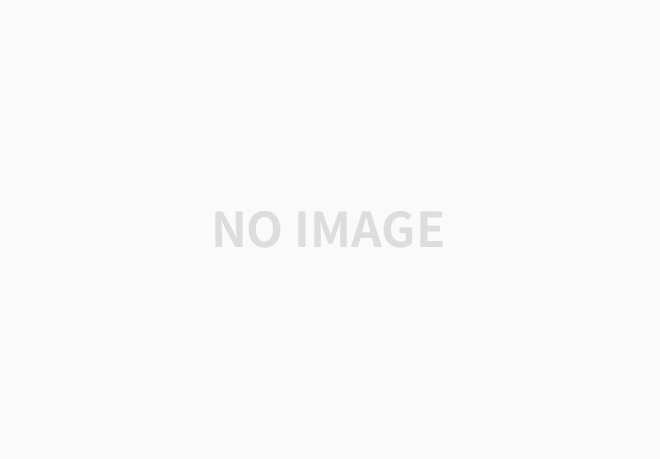
변수 출력 예제코드2
name2는 name를 참조하고 있음으로 name의 값을 변경하면 name2도 변경된다.
1
2
3
4
5
6
|
name="jo"
name2=name
name[0]='S'
p "#{name}"
p "#{name2}"
|
cs |
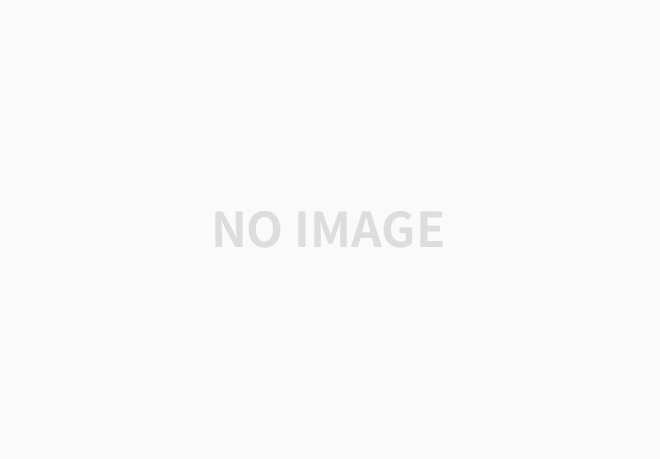
변수 출력 예제코드3
다른 변수 값을 참조할때 참조한 변수의 값이 변경되어도 영향을 받지 않을라면 "dup"를 사용한다.
1
2
3
4
5
6
|
name="jo"
name2=name.dup
name[0]='S'
p "#{name}"
p "#{name2}"
|
cs |
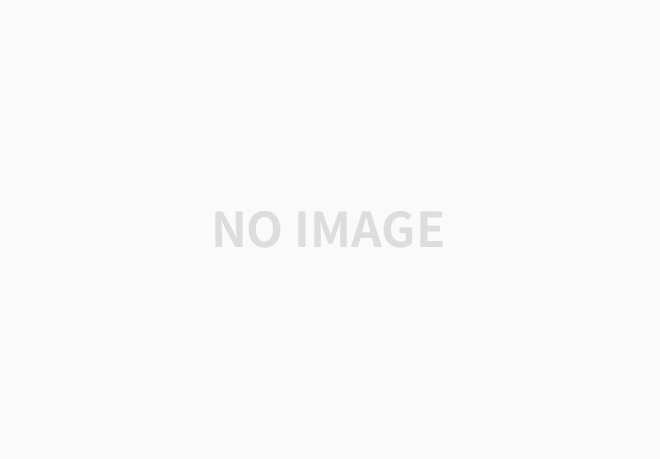
클래스
기본형식 : class 클래스명 end
인스턴스 생성 형식 : 인스턴스명 = 클래스명.new
클래스, 인스턴스 선언 사용 예제코드
initialize는 클래스의 처음이며 변수?를 초기화 해주는 메소드이다..
"p"와 "puts"의 차이도 확인해 둔다.
1
2
3
4
5
6
7
8
9
|
class Test
def initialize(str,num)
@str=str
@num=num
end
end
test1=Test.new("jo",1)
p test1
puts test1 |
cs |

클래스, 인스턴스 선언 사용 예제코드2
to_s 메소드를 이용하면 출력 결과를 예쁘게 나타낼 수 있다.
이때도 "p"와 "puts"의 차이를 확인해 둔다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
class Test
def initialize(str,num)
@str=str
@num=num
end
def to_s
"name : #{@str}, num : #{@num}"
end
end
test1=Test.new("jo",1)
p test1
puts test1
|
cs |
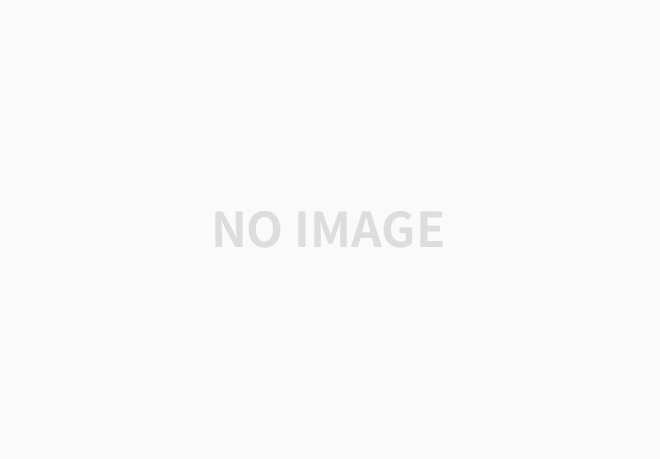
클래스, 인스턴스 선언 사용 예제코드3
각 변수를 따로 호출이나 사용하고 싶으면 그에 맞는 메소드를 작성해줘야 한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
class Test
def initialize(str,num)
@str=str
@num=num
end
def str
@str
end
end
test1=Test.new("jo",1)
puts "#{test1.str}"
|
cs |
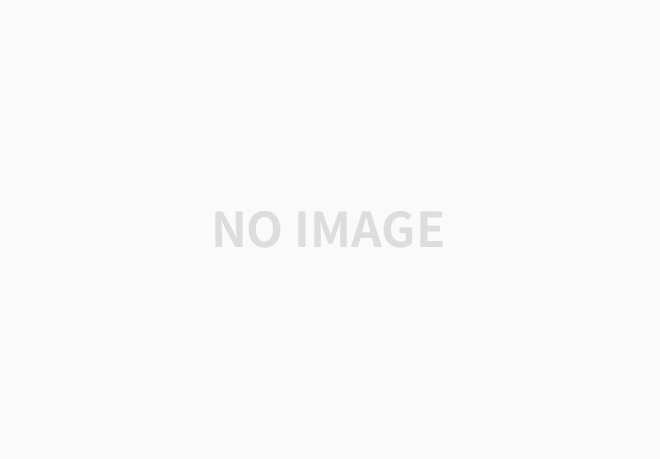
해시
해시는 파이썬의 딕셔너리처럼 키와 값을 가지는 형식이다.
해시 예제코드1
키를 문자열로 사용한 예제
1
2
3
4
5
6
|
test ={'A'=>'a', 'B'=>'b', 'C'=>'c'}
p test
p test['A']
p test['B']
p test[10]='j'
p test
|
cs |
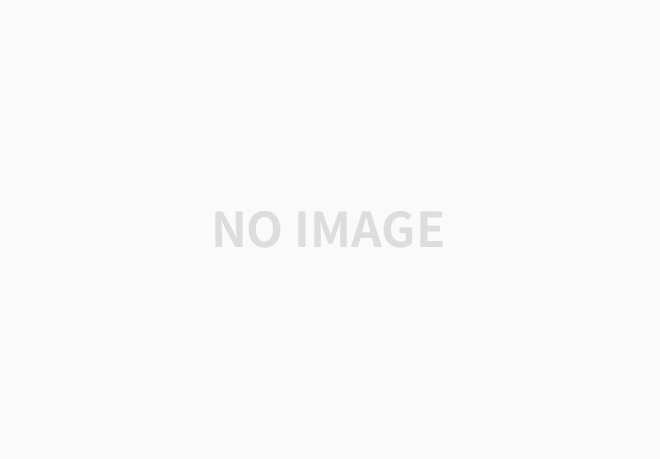
키를 상수로 사용한 예제
1
2
3
4
5
6
7
|
test ={:A=>'a', :B=>'b', :C=>'c'}
# test ={A:'a', B:'b', C:'c'}
p test
p test[:A]
p test[:B]
p test[10]='j'
p test
|
cs |
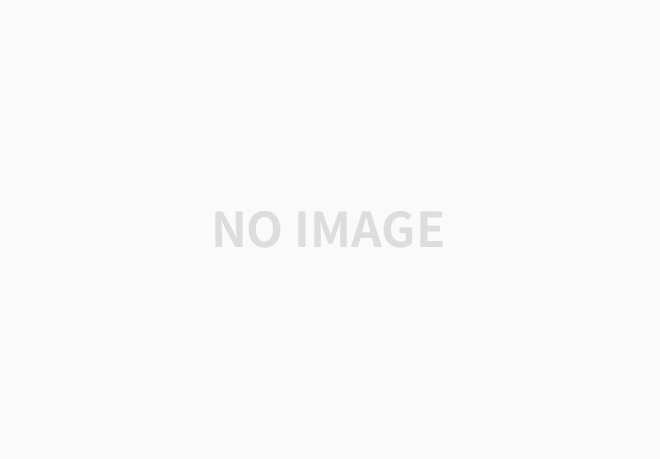
'프로그래밍 > Ruby' 카테고리의 다른 글
[Ruby require net/http] Basic_auth, Digest_auth, proxy setting (0) | 2020.08.25 |
---|---|
[Ruby require net/http] URI 파싱, GET, POST, header setting (0) | 2020.08.25 |
기초 4 (파일 입출력) (0) | 2020.08.25 |
기초 3 (블록, 변수 대입, 예외처리(rescue), catch/throw) (0) | 2020.08.18 |
기초 (인자, 읽기 쓰기, 메소드, 배열, 키와 값, nil, 상수(심볼), IF문, While문) (0) | 2020.08.14 |